This content originally appeared on DEV Community and was authored by Abdulkabir sultan
What is object-oriented programming?
Object-oriented programming (OOP) is a programming paradigm used by developers to structure software applications into reusable pieces of code and blueprints using objects. OOP focuses on the objects that developers want to manipulate rather than the logic required to manipulate them. This approach to programming is well-suited for programs that are large, complex, and actively updated or maintained.
Popular programming languages which uses object oriented programming include Java, Python, c# and C++.
In this article, we would provide an overview of the basic concepts of OOP. We'll also look at the four major principles of OOP.
Building Blocks or structure of OOP
The building blocks of object-oriented programming include classes, objects,methods, attributes.
1. Class:
- A class is a blueprint or a template for creating objects that share common characteristics and behaviors.
- It defines the properties and methods that the objects of the class will have.
- Example: A class can be "Person" which defines the properties and methods of a person.
2. Object:
- An object is an instance of a class. It is created using the blueprint provided by the class.
- It has its own unique set of values for the properties defined by the class.
- Example: An object can be "John" which is an instance of the "Person" class.
3. Method:
- A method is a function that belongs to a class or an object. It defines the behavior of the object of the class.
- It can manipulate the properties of the object or perform some operation using the properties.
- Example: A method of the "Person" class can be "say_hello()" which will print a greeting message.
4. Attribute:
- An attribute is a characteristic of a class or an object. It defines the state of the object of the class.
- It can be a variable that stores some value or a constant that defines a property.
- Example: An attribute of the "Person" class can be "age" which stores the age of the person object.
What are the main principles of OOP?
Object-oriented programming is based on the following principles:
- Encapsulation
- Abstraction
- Polymorphism
- Inheritance
1. Encapsulation:
Encapsulation is the process of hiding the implementation details of a class from the outside world.
It protects the data and methods of a class from being accessed or modified by other code.
Example
The "age" attribute of the "Person" class can be made private, so it can only be accessed or modified from within the class meaning, it is encapsulated within the class.
2. Abstraction:
Abstraction is the process of hiding complex implementation details and exposing only essential features of an object.
It provides simplicity and ease of use for the user.
Example
The "say_hello()" method of the "Person" class is an abstraction, as it hides the complexity of the greeting message and only exposes the essential feature of greeting someone.
3. Polymorphism
Polymorphism is the ability of objects of different classes to be treated as if they were objects of the same class.
It allows for flexibility and code reuse.
Example
The "say_hello()" method can be implemented in different ways for different objects (e.g., "Employee" object, "Customer" object) while still being treated as a "Person" object.
4. Inheritance:
Inheritance is the process of creating a new class that inherits properties and methods from an existing class (i.e., parent class).
It allows for code reuse and adding new functionality to the new class.
Example
A new class "Student" can inherit properties and methods from the "Person" class, such as "name" and "say_hello()", while adding new properties and methods specific to the "Student" class (e.g., "major" attribute, "study()" method).
What are the benefits of OOP?
Code reusability: OOP allows for code reuse, which means that code can be written once and reused in different parts of the program. This saves time and effort in programming.
Easy maintenance: it promotes code maintenance by making code more modular and organized. Changes can be made to individual objects without affecting the rest of the program, making it easier to debug and fix errors.
Encapsulation: Object oriented programming promotes encapsulation, which means that the implementation details of an object are hidden from the outside world. This improves code security by preventing external code from interfering with the internal workings of an object.
Flexibility: It allows for flexibility, which means that objects can be easily modified or extended without affecting other parts of the program. This makes it easier to adapt code to changing requirements or new features.
In conclusion, Object-Oriented Programming (OOP) is a programming paradigm that allows for the creation of modular, reusable, and scalable code. It provides several benefits, including code reusability and easy maintenance, it promotes the creation of objects that encapsulate data and behavior, making it easier to model real-world entities and interactions. By dividing code into smaller, more manageable pieces. OOP is widely used in modern software development and has become a fundamental tool for programmers to create efficient, robust, and scalable applications.
This content originally appeared on DEV Community and was authored by Abdulkabir sultan
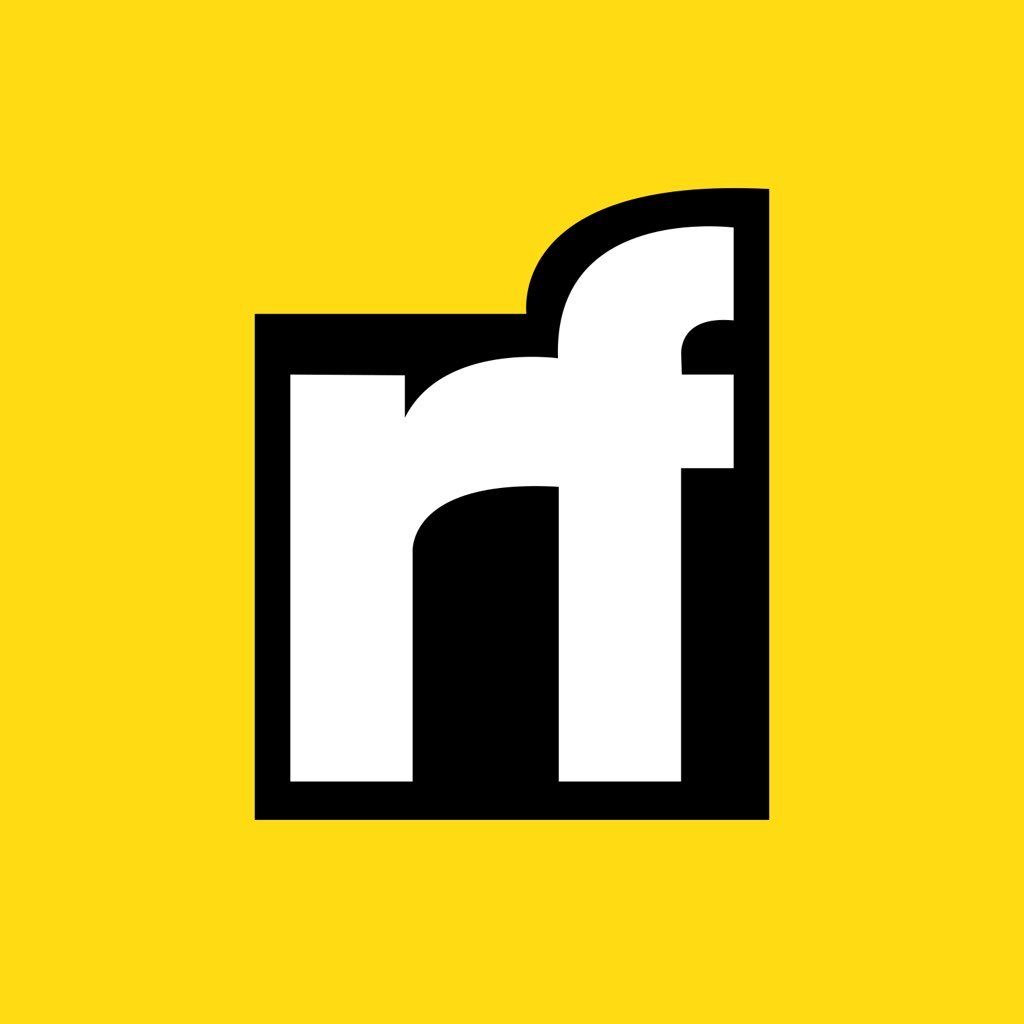
Abdulkabir sultan | Sciencx (2023-04-27T00:53:21+00:00) Object-oriented programming (OOP). Retrieved from https://www.scien.cx/2023/04/27/object-oriented-programming-oop-2/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.