This content originally appeared on Level Up Coding - Medium and was authored by Onur Derman
Smaller Interfaces, Better Software: How to Apply Interface Segregation Principle for Smarter Code Design
The Interface Segregation Principle (ISP) is one of the five principles of SOLID design that help developers write clean and maintainable code. The ISP states that clients should not be forced to depend on methods that they do not use. In other words, interfaces should be small and focused, rather than large and general
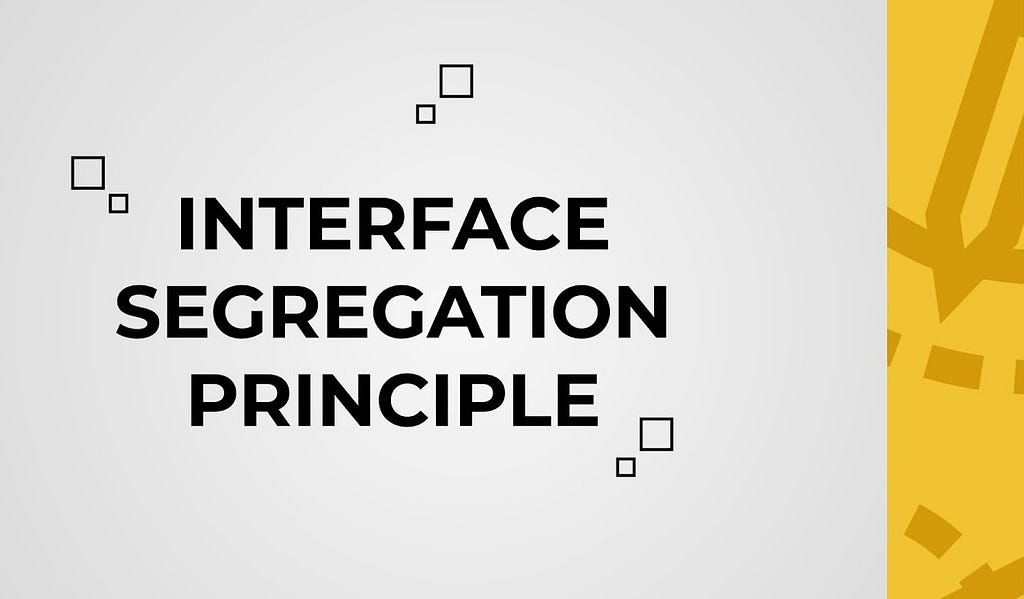
Why is the ISP important?
The ISP helps to avoid the problems that arise from having fat interfaces, which are interfaces that have too many methods or responsibilities. Some of these problems are:
- Increased coupling: When a client depends on a fat interface, it also depends on all the methods and types that the interface uses, even if they are irrelevant to the client. This creates unnecessary dependencies and makes the code harder to change or test.
- Reduced cohesion: When an interface has too many methods or responsibilities, it becomes unclear what its main purpose is. This makes the code less readable and understandable.
- Violation of the Single Responsibility Principle: When an interface has too many methods or responsibilities, it is likely that some of them belong to different domains or levels of abstraction. This means that the interface is doing more than one thing and violates the Single Responsibility Principle, which states that a class or interface should have only one reason to change.
How to apply the ISP?
The ISP can be applied by following these steps:
- Identify the clients that use an interface and their needs.
- Split the interface into smaller and more specific interfaces that only contain the methods that each client needs.
- Make the clients depend on the smaller interfaces instead of the fat interface.
C# Code Examples
Let’s see an example of how to apply the ISP in C#. Suppose we have a fat interface called IEmployee that represents an employee in a company:
public interface IEmployee
{
string Name { get; set; }
string Department { get; set; }
decimal Salary { get; set; }
void Hire();
void Fire();
void Promote();
void Demote();
void Transfer(string newDepartment);
void Work();
}
This interface has too many methods and responsibilities. It mixes the concepts of hiring, firing, promoting, demoting, transferring, and working. Not all clients that use this interface need all these methods. For example, a payroll system only needs to access the name, department, and salary of an employee, but not to hire or fire them. A human resources system needs to hire and fire employees, but not to make them work.
To apply the ISP, we can split this interface into smaller and more specific interfaces:
public interface IEmployee
{
string Name { get; set; }
string Department { get; set; }
decimal Salary { get; set; }
}
public interface IEmployeeManagement
{
void Hire(IEmployee employee);
void Fire(IEmployee employee);
void Promote(IEmployee employee);
void Demote(IEmployee employee);
void Transfer(IEmployee employee, string newDepartment);
}
public interface IEmployeeWork
{
void Work();
}
Now we have three interfaces: IEmployee, which contains the basic properties of an employee; IEmployeeManagement, which contains the methods related to managing employees; and IEmployeeWork, which contains the method related to working. Each interface has a clear and focused responsibility.
We can then make the clients depend on the smaller interfaces instead of the fat interface. For example:
public class PayrollSystem
{
public void PayEmployees(IEnumerable<IEmployee> employees)
{
foreach (var employee in employees)
Console.WriteLine($"Paying {employee.Name} from {employee.Department} ${employee.Salary}");
}
}
public class HumanResourcesSystem
{
private readonly IEmployeeManagement _employeeManagement;
public HumanResourcesSystem(IEmployeeManagement employeeManagement)
{
_employeeManagement = employeeManagement;
}
public void HireEmployee(IEmployee employee)
{
_employeeManagement.Hire(employee);
Console.WriteLine($"Hired {employee.Name} to {employee.Department}");
}
public void FireEmployee(IEmployee employee)
{
_employeeManagement.Fire(employee);
Console.WriteLine($"Fired {employee.Name} from {employee.Department}");
}
// Other methods omitted for brevity
}
public class WorkSystem
{
public void MakeEmployeesWork(IEnumerable<IEmployeeWork> employees)
{
foreach (var employee in employees)
employee.Work();
}
}
By applying the ISP, we have reduced the coupling between the clients and the interfaces, increased the cohesion of the interfaces, and respected the Single Responsibility Principle. The code is now more clean and maintainable.
I appreciate your participation and hope that my article has provided you with valuable insights. Thank you for taking the time to read it thus far.
Smaller Interfaces, Better Software: How to Apply Interface Segregation Principle for Smarter Code… was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Onur Derman
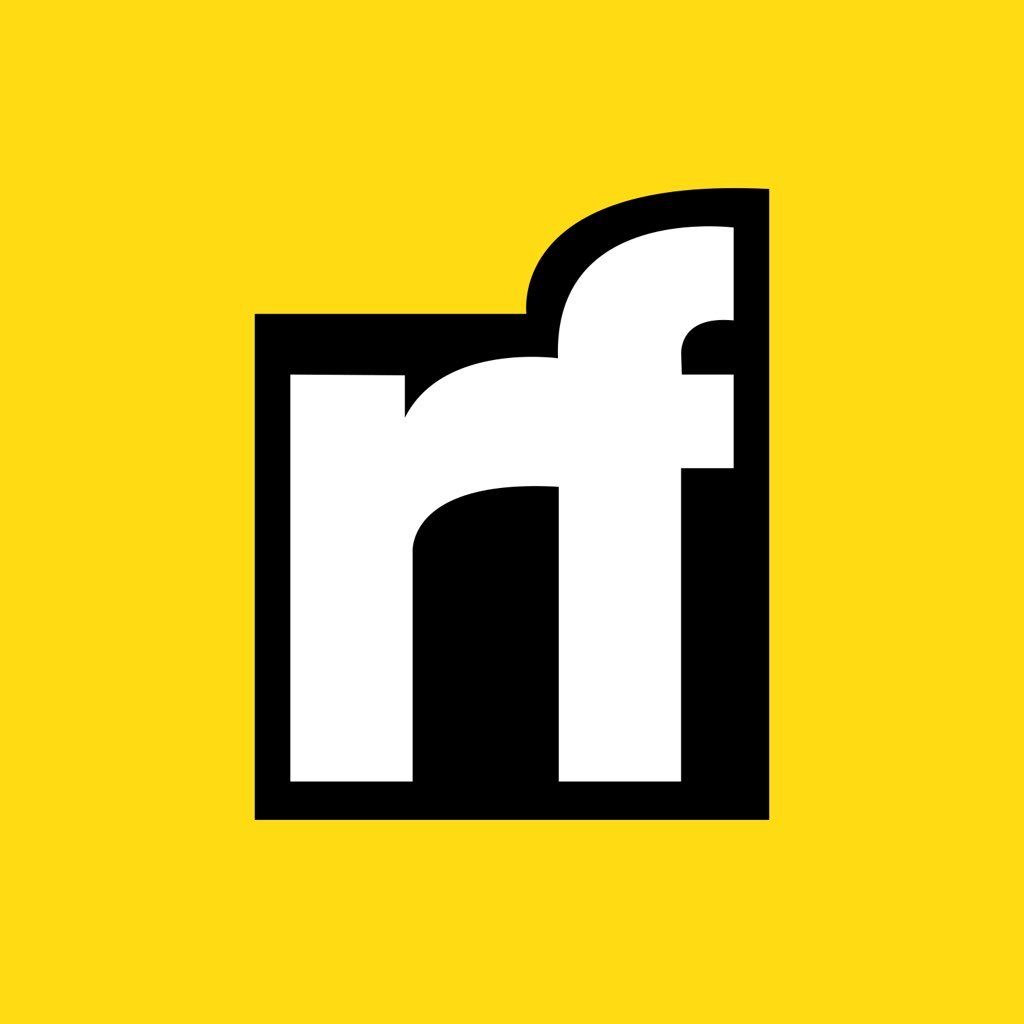
Onur Derman | Sciencx (2023-05-14T15:08:13+00:00) Smaller Interfaces, Better Software: How to Apply Interface Segregation Principle for Smarter Code…. Retrieved from https://www.scien.cx/2023/05/14/smaller-interfaces-better-software-how-to-apply-interface-segregation-principle-for-smarter-code/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.